How to Deploy an s3 bucket using Terraform via GitHub Actions in 6 steps
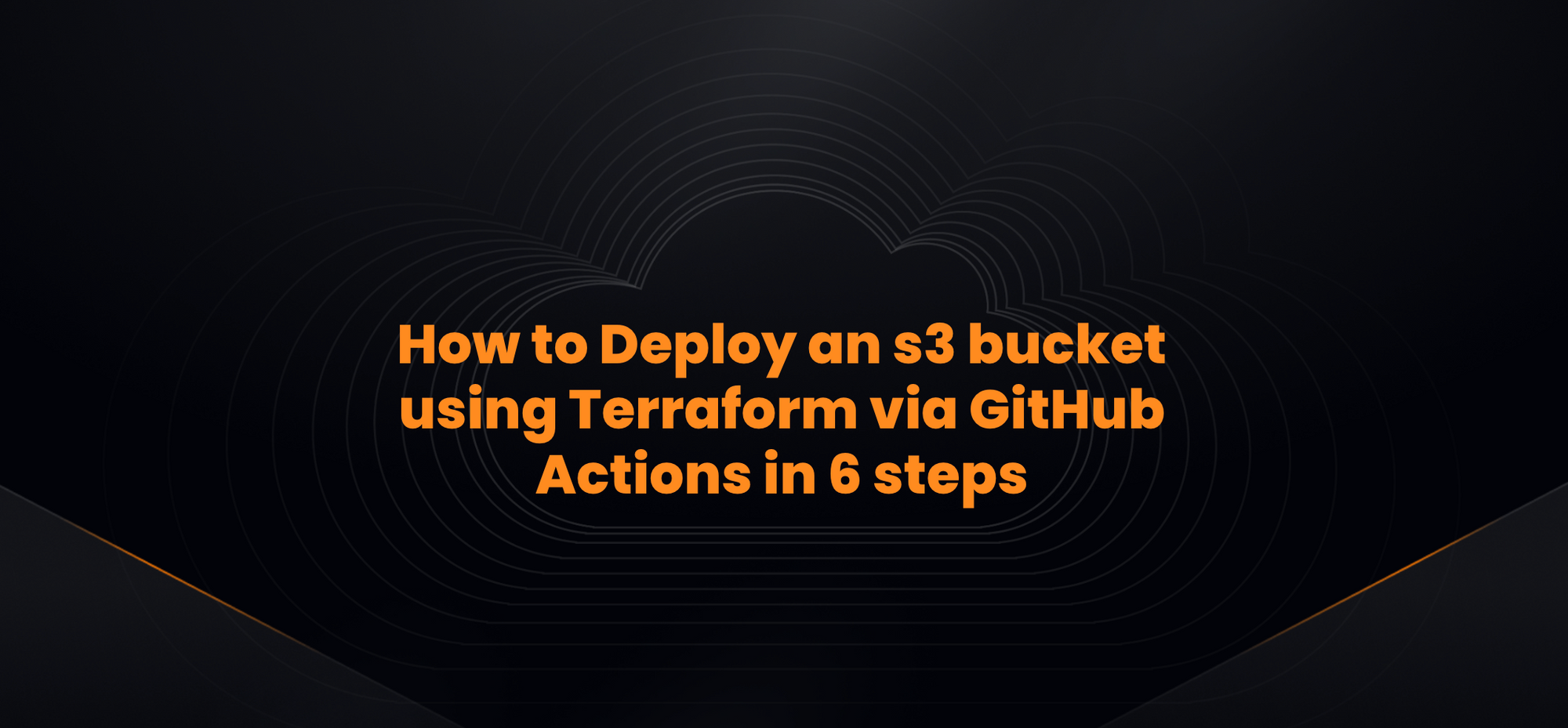
Deploying an Amazon S3 bucket using Terraform via GitHub Actions allows you to automate your infrastructure as code process. Here's a step-by-step tutorial:
1. Setting up your Terraform Configuration
provider "aws" {
region = "us-west-1"
version = "~> 3.0"
}
resource "aws_s3_bucket" "my_bucket" {
bucket = "my-unique-bucket-name"
acl = "private"
}
Ensure you have the necessary providers configured, and specify the S3 bucket configuration.
2. Set Up GitHub Repository
- Create a new repository on GitHub.
- Push the above Terraform configuration to the repository.
3. AWS Credentials
You'll need AWS credentials (AWS_ACCESS_KEY_ID
and AWS_SECRET_ACCESS_KEY
) for Terraform to interact with AWS.
Never push these secrets to GitHub or expose them in logs. Instead, use GitHub Secrets:
- Go to your GitHub repository.
- Click on the
Settings
tab. - Go to the
Secrets
section. - Add
AWS_ACCESS_KEY_ID
andAWS_SECRET_ACCESS_KEY
.
4. GitHub Actions Configuration
In your repository, create a new file: .github/workflows/terraform.yml
name: 'Terraform'
on:
push:
branches:
- main
jobs:
terraform:
name: 'Terraform'
runs-on: ubuntu-latest
steps:
- name: Checkout
uses: actions/checkout@v2
- name: Set up Terraform
uses: hashicorp/setup-terraform@v1
- name: Terraform Initialize
env:
AWS_ACCESS_KEY_ID: ${{ secrets.AWS_ACCESS_KEY_ID }}
AWS_SECRET_ACCESS_KEY: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
run: terraform init
- name: Terraform Validate
run: terraform validate
- name: Terraform Plan
env:
AWS_ACCESS_KEY_ID: ${{ secrets.AWS_ACCESS_KEY_ID }}
AWS_SECRET_ACCESS_KEY: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
run: terraform plan
- name: Terraform Apply
env:
AWS_ACCESS_KEY_ID: ${{ secrets.AWS_ACCESS_KEY_ID }}
AWS_SECRET_ACCESS_KEY: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
run: terraform apply -auto-approve
This workflow does the following:
- Checks out your repository.
- Sets up Terraform.
- Initializes Terraform.
- Validates Terraform configurations.
- Creates a Terraform plan.
- Applies the Terraform configuration.
5. Commit and Push
Once you've set up the GitHub Actions workflow, commit and push the .github/workflows/terraform.yml
file to your GitHub repository.
6. Verify
After pushing the GitHub Actions configuration:
- Go to the
Actions
tab in your GitHub repository. - You should see the Terraform workflow running.
- Once it's completed, you can verify in AWS that your S3 bucket has been created.
Important Notes:
- Always ensure that your AWS credentials are securely stored and not hard-coded or exposed.
- If you're working on a team or in a production environment, you might want to add approval steps before the
terraform apply
to ensure changes are reviewed. - Ensure your bucket name is unique globally.
- Adjust the AWS region in the Terraform configuration if needed.
- Consider using state backends like S3 or Terraform Cloud to manage and store your Terraform state.
This is a basic tutorial, and there are many nuances to consider for production-grade infrastructure deployment, including managing Terraform state, handling secrets, and more.
If you are considering using GitHub Actions for production-grade infrastructure deployment - Digger simplifies running Terraform & OpenTofu in the CI/CD system of your choice. Digger has:
- Private runners by default - no sharing of secrets with a 3rd party
- Is scalable & reliable - Digger reuses your existing CI/CD system for compute
- Faster Deployments - Digger has parallel runs enabled on all plans
- Easy to get started - No need to host and maintain an extra server.
- Audit Trails - Digger Maintains an audit trail of all deployments & changes.
- Policies - Enforce project and organisation level policies (Via OPA) for compliance.
- RBAC - Control who can view, modify, and deploy infrastructure based on their role.
- Single Sign-On (SSO) via SAML - User authentication and access management with SSO through SAML integration.